PHPCS: WordPress
Personal documentation for the sniffs available in the PHP Code Sniffer coding standards to help build an own rulesets and search for error explanations.
Generic.Classes.DuplicateClassName
| [ref]
Class and Interface names should be unique in a project. They should never be duplicated.
Valid: A unique class name. | Invalid: A class duplicated (including across multiple files). |
---|---|
|
|
Generic.Classes.OpeningBraceSameLine
| [ref]
The opening brace of a class must be on the same line after the definition and must be the last thing on that line.
Valid: Opening brace on the same line. | Invalid: Opening brace on the next line. |
---|---|
|
|
Valid: Opening brace is the last thing on the line. | Invalid: Opening brace not last thing on the line. |
---|---|
|
|
Generic.CodeAnalysis.AssignmentInCondition
| [ref]
Variable assignments should not be made within conditions.
Valid: A variable comparison being executed within a condition. | Invalid: A variable assignment being made within a condition. |
---|---|
|
|
Generic.CodeAnalysis.EmptyPHPStatement
| [ref]
Empty PHP tags are not allowed.
Valid: There is at least one statement inside the PHP tag pair. | Invalid: There is no statement inside the PHP tag pair. |
---|---|
|
|
Valid: There is no superfluous semi-colon after a PHP statement. | Invalid: There are one or more superfluous semi-colons after a PHP statement. |
---|---|
|
|
Generic.CodeAnalysis.EmptyStatement
| [ref]
Control Structures must have at least one statement inside of the body.
Valid: There is a statement inside the control structure. | Invalid: The control structure has no statements. |
---|---|
|
|
Generic.CodeAnalysis.ForLoopShouldBeWhileLoop
| [ref]
For loops that have only a second expression (the condition) should be converted to while loops.
Valid: A for loop is used with all three expressions. | Invalid: A for loop is used without a first or third expression. |
---|---|
|
|
Generic.CodeAnalysis.ForLoopWithTestFunctionCall
| [ref]
For loops should not call functions inside the test for the loop when they can be computed beforehand.
Valid: A for loop that determines its end condition before the loop starts. | Invalid: A for loop that unnecessarily computes the same value on every iteration. |
---|---|
|
|
Generic.CodeAnalysis.JumbledIncrementer
| [ref]
Incrementers in nested loops should use different variable names.
Valid: Two different variables being used to increment. | Invalid: Inner incrementer is the same variable name as the outer one. |
---|---|
|
|
Generic.CodeAnalysis.RequireExplicitBooleanOperatorPrecedence
| [ref]
Forbids mixing different binary boolean operators (&&, ||, and, or, xor) within a single expression without making precedence clear using parentheses.
Valid: Making precedence clear with parentheses. | Invalid: Not using parentheses. |
---|---|
|
|
Generic.CodeAnalysis.UnconditionalIfStatement
| [ref]
If statements that are always evaluated should not be used.
Valid: An if statement that only executes conditionally. | Invalid: An if statement that is always performed. |
---|---|
|
|
Valid: An if statement that only executes conditionally. | Invalid: An if statement that is never performed. |
---|---|
|
|
Generic.CodeAnalysis.UnnecessaryFinalModifier
| [ref]
Methods should not be declared final inside of classes that are declared final.
Valid: A method in a final class is not marked final. | Invalid: A method in a final class is also marked final. |
---|---|
|
|
Generic.CodeAnalysis.UnusedFunctionParameter
| [ref]
All parameters in a functions signature should be used within the function.
Valid: All the parameters are used. | Invalid: One of the parameters is not being used. |
---|---|
|
|
Generic.CodeAnalysis.UselessOverridingMethod
| [ref]
Methods should not be defined that only call the parent method.
Valid: A method that extends functionality on a parent method. | Invalid: An overriding method that only calls the parent. |
---|---|
|
|
Generic.Commenting.DocComment
| [ref]
Generic.ControlStructures.InlineControlStructure
| [ref]
Control Structures should use braces.
Valid: Braces are used around the control structure. | Invalid: No braces are used for the control structure.. |
---|---|
|
|
Generic.Files.ByteOrderMark
| [ref]
Byte Order Marks that may corrupt your application should not be used. These include 0xefbbbf (UTF-8), 0xfeff (UTF-16 BE) and 0xfffe (UTF-16 LE).
Generic.Files.LineEndings
| [ref]
Unix-style line endings are preferred ("\n" instead of "\r\n").
Generic.Files.OneObjectStructurePerFile
| [ref]
There should only be one class or interface or trait defined in a file.
Valid: Only one object structure in the file. | Invalid: Multiple object structures defined in one file. |
---|---|
|
|
Generic.Formatting.DisallowMultipleStatements
| [ref]
Multiple statements are not allowed on a single line.
Valid: Two statements are spread out on two separate lines. | Invalid: Two statements are combined onto one line. |
---|---|
|
|
Generic.Formatting.MultipleStatementAlignment
| [ref]
There should be one space on either side of an equals sign used to assign a value to a variable. In the case of a block of related assignments, more space may be inserted to promote readability.
Equals signs aligned | Not aligned; harder to read |
---|---|
|
|
Equals signs aligned; only one space after longest var name | Two spaces after longest var name |
---|---|
|
|
Equals signs aligned | Equals signs not aligned |
---|---|
|
|
Generic.Formatting.SpaceAfterCast
| [ref]
Exactly one space is allowed after a cast.
Valid: A cast operator is followed by one space. | Invalid: A cast operator is not followed by whitespace. |
---|---|
|
|
Generic.Functions.CallTimePassByReference
| [ref]
Call-time pass-by-reference is not allowed. It should be declared in the function definition.
Valid: Pass-by-reference is specified in the function definition. | Invalid: Pass-by-reference is done in the call to a function. |
---|---|
|
|
Generic.Functions.FunctionCallArgumentSpacing
| [ref]
Function arguments should have one space after a comma, and single spaces surrounding the equals sign for default values.
Valid: Single spaces after a comma. | Invalid: No spaces after a comma. |
---|---|
|
|
Valid: Single spaces around an equals sign in function declaration. | Invalid: No spaces around an equals sign in function declaration. |
---|---|
|
|
Generic.Functions.OpeningFunctionBraceKernighanRitchie
| [ref]
Function declarations follow the "Kernighan/Ritchie style". The function brace is on the same line as the function declaration. One space is required between the closing parenthesis and the brace.
Valid: brace on same line | Invalid: brace on next line |
---|---|
|
|
Generic.NamingConventions.UpperCaseConstantName
| [ref]
Constants should always be all-uppercase, with underscores to separate words.
Valid: all uppercase | Invalid: mixed case |
---|---|
|
|
Generic.PHP.BacktickOperator
| [ref]
Disallow the use of the backtick operator for execution of shell commands.
Generic.PHP.DeprecatedFunctions
| [ref]
Deprecated functions should not be used.
Valid: A non-deprecated function is used. | Invalid: A deprecated function is used. |
---|---|
|
|
Generic.PHP.DisallowAlternativePHPTags
| [ref]
Always use <?php ?> to delimit PHP code, do not use the ASP <% %> style tags nor the <script language="php"></script> tags. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.DisallowShortOpenTag
| [ref]
Always use <?php ?> to delimit PHP code, not the <? ?> shorthand. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.DiscourageGoto
| [ref]
Discourage the use of the PHP goto
language construct.
Generic.PHP.ForbiddenFunctions
| [ref]
The forbidden functions sizeof() and delete() should not be used.
Valid: count() is used in place of sizeof(). | Invalid: sizeof() is used. |
---|---|
|
|
Generic.PHP.LowerCaseConstant
| [ref]
The true, false and null constants must always be lowercase.
Valid: lowercase constants | Invalid: uppercase constants |
---|---|
|
|
Generic.PHP.LowerCaseKeyword
| [ref]
All PHP keywords should be lowercase.
Valid: Lowercase array keyword used. | Invalid: Non-lowercase array keyword used. |
---|---|
|
|
Generic.PHP.LowerCaseType
| [ref]
All PHP types used for parameter type and return type declarations should be lowercase.
Valid: Lowercase type declarations used. | Invalid: Non-lowercase type declarations used. |
---|---|
|
|
Valid: Lowercase type used. | Invalid: Non-lowercase type used. |
---|---|
|
|
Generic.PHP.Syntax
| [ref]
The code should use valid PHP syntax.
Valid: No PHP syntax errors. | Invalid: Code contains PHP syntax errors. |
---|---|
|
|
Generic.Strings.UnnecessaryStringConcat
| [ref]
Strings should not be concatenated together.
Valid: A string can be concatenated with an expression. | Invalid: Strings should not be concatenated together. |
---|---|
|
|
Generic.VersionControl.GitMergeConflict
| [ref]
Generic.WhiteSpace.ArbitraryParenthesesSpacing
| [ref]
Arbitrary sets of parentheses should have no spaces inside.
Valid: no spaces on the inside of a set of arbitrary parentheses. | Invalid: spaces or new lines on the inside of a set of arbitrary parentheses. |
---|---|
|
|
Generic.WhiteSpace.DisallowSpaceIndent
| [ref]
Tabs should be used for indentation instead of spaces.
Generic.WhiteSpace.IncrementDecrementSpacing
| [ref]
There should be no whitespace between variables and increment/decrement operators.
Valid: No whitespace between variables and increment/decrement operators. | Invalid: Whitespace between variables and increment/decrement operators. |
---|---|
|
|
Generic.WhiteSpace.LanguageConstructSpacing
| [ref]
Language constructs that can be used without parentheses, must have a single space between the language construct keyword and its content.
Valid: Single space after language construct. | Invalid: No space, more than one space or newline after language construct. |
---|---|
|
|
Valid: Single space between yield and from. | Invalid: No space, more than one space or newline between yield and from. |
---|---|
|
|
Generic.WhiteSpace.ScopeIndent
| [ref]
Indentation for control structures, classes, and functions should be 4 spaces per level.
Valid: 4 spaces are used to indent a control structure. | Invalid: 8 spaces are used to indent a control structure. |
---|---|
|
|
Generic.WhiteSpace.SpreadOperatorSpacingAfter
| [ref]
There should be no space between the spread operator and the variable/function call it applies to.
Valid: No space between the spread operator and the variable/function call it applies to. | Invalid: space found between the spread operator and the variable/function call it applies to. |
---|---|
|
|
Modernize.FunctionCalls.Dirname
| [ref]
PHP >= 5.3: Usage of dirname(FILE) can be replaced with DIR.
Valid: Using __DIR__. | Invalid: dirname(__FILE__). |
---|---|
|
|
Valid: Using dirname() with the $levels parameter. | Invalid: Nested function calls to dirname(). |
---|---|
|
|
NormalizedArrays.Arrays.ArrayBraceSpacing
| [ref]
There should be no space between the "array" keyword and the array open brace.
Valid: No space between the keyword and the open brace. | Invalid: Space between the keyword and the open brace. |
---|---|
|
|
Valid: No space between the braces. | Invalid: Space between the braces. |
---|---|
|
|
Valid: No space on the inside of the braces. | Invalid: Space on the inside of the braces. |
---|---|
|
|
Valid: One new line after the open brace and before the close brace. | Invalid: No new lines after the open brace and/or before the close brace. |
---|---|
|
|
NormalizedArrays.Arrays.CommaAfterLast
| [ref]
For single-line arrays, there should be no comma after the last array item.
However, for multi-line arrays, there *should* be a comma after the last array item.
Valid: Single-line array without a comma after the last item | Invalid: Single-line array with a comma after the last item |
---|---|
|
|
Valid: Multi-line array with a comma after the last item | Invalid: Multi-line array without a comma after the last item |
---|---|
|
|
PEAR.Files.IncludingFile
| [ref]
Anywhere you are unconditionally including a class file, use require_once. Anywhere you are conditionally including a class file (for example, factory methods), use include_once. Either of these will ensure that class files are included only once. They share the same file list, so you don't need to worry about mixing them - a file included with require_once will not be included again by include_once. Note that include_once and require_once are statements, not functions. Parentheses should not surround the subject filename.
Valid: used as statement | Invalid: used as function |
---|---|
|
|
PEAR.Functions.FunctionCallSignature
| [ref]
Functions should be called with no spaces between the function name, the opening parenthesis, and the first parameter; and no space between the last parameter, the closing parenthesis, and the semicolon.
Valid: spaces between parameters | Invalid: additional spaces used |
---|---|
|
|
PEAR.NamingConventions.ValidClassName
| [ref]
Classes should be given descriptive names. Avoid using abbreviations where possible. Class names should always begin with an uppercase letter. The PEAR class hierarchy is also reflected in the class name, each level of the hierarchy separated with a single underscore.
Examples of valid class names | Examples of invalid class names |
---|---|
|
|
PSR2.Classes.ClassDeclaration
| [ref]
There should be exactly 1 space between the abstract or final keyword and the class keyword and between the class keyword and the class name. The extends and implements keywords, if present, must be on the same line as the class name. When interfaces implemented are spread over multiple lines, there should be exactly 1 interface mentioned per line indented by 1 level. The closing brace of the class must go on the first line after the body of the class and must be on a line by itself.
Valid: Correct spacing around class keyword. | Invalid: 2 spaces used around class keyword. |
---|---|
|
|
PSR2.Classes.PropertyDeclaration
| [ref]
Property names should not be prefixed with an underscore to indicate visibility. Visibility should be used to declare properties rather than the var keyword. Only one property should be declared within a statement. The static declaration must come after the visibility declaration.
Valid: Correct property naming. | Invalid: An underscore prefix used to indicate visibility. |
---|---|
|
|
Valid: Visibility of property declared. | Invalid: Var keyword used to declare property. |
---|---|
|
|
Valid: One property declared per statement. | Invalid: Multiple properties declared in one statement. |
---|---|
|
|
Valid: If declared as static, the static declaration must come after the visibility declaration. | Invalid: Static declaration before the visibility declaration. |
---|---|
|
|
PSR2.ControlStructures.ElseIfDeclaration
| [ref]
PHP's elseif keyword should be used instead of else if.
Valid: Single word elseif keyword used. | Invalid: Separate else and if keywords used. |
---|---|
|
|
PSR2.ControlStructures.SwitchDeclaration
| [ref]
Case statements should be indented 4 spaces from the switch keyword. It should also be followed by a space. Colons in switch declarations should not be preceded by whitespace. Break statements should be indented 4 more spaces from the case statement. There must be a comment when falling through from one case into the next.
Valid: Case statement indented correctly. | Invalid: Case statement not indented 4 spaces. |
---|---|
|
|
Valid: Case statement followed by 1 space. | Invalid: Case statement not followed by 1 space. |
---|---|
|
|
Valid: Colons not prefixed by whitespace. | Invalid: Colons prefixed by whitespace. |
---|---|
|
|
Valid: Break statement indented correctly. | Invalid: Break statement not indented 4 spaces. |
---|---|
|
|
Valid: Comment marking intentional fall-through. | Invalid: No comment marking intentional fall-through. |
---|---|
|
|
PSR2.Files.ClosingTag
| [ref]
Checks that the file does not end with a closing tag.
Valid: Closing tag not used. | Invalid: Closing tag used. |
---|---|
|
|
PSR2.Files.EndFileNewline
| [ref]
PHP Files should end with exactly one newline.
PSR2.Methods.FunctionClosingBrace
| [ref]
Checks that the closing brace of a function goes directly after the body.
Valid: Closing brace directly follows the function body. | Invalid: Blank line between the function body and the closing brace. |
---|---|
|
|
PSR2.Methods.MethodDeclaration
| [ref]
Method names should not be prefixed with an underscore to indicate visibility. The static keyword, when present, should come after the visibility declaration, and the final and abstract keywords should come before.
Valid: Correct method naming. | Invalid: An underscore prefix used to indicate visibility. |
---|---|
|
|
Valid: Correct ordering of method prefixes. | Invalid: static keyword used before visibility and final used after. |
---|---|
|
|
PSR2.Namespaces.NamespaceDeclaration
| [ref]
There must be one blank line after the namespace declaration.
Valid: One blank line after the namespace declaration. | Invalid: No blank line after the namespace declaration. |
---|---|
|
|
PSR12.Classes.ClassInstantiation
| [ref]
When instantiating a new class, parenthesis MUST always be present even when there are no arguments passed to the constructor.
Valid: Parenthesis used. | Invalid: Parenthesis not used. |
---|---|
|
|
PSR12.Files.FileHeader
| [ref]
PSR12.Functions.NullableTypeDeclaration
| [ref]
In nullable type declarations there MUST NOT be a space between the question mark and the type.
Valid: no whitespace used. | Invalid: superfluous whitespace used. |
---|---|
|
|
Valid: no unexpected characters. | Invalid: unexpected characters used. |
---|---|
|
|
PSR12.Functions.ReturnTypeDeclaration
| [ref]
For function and closure return type declarations, there must be one space after the colon followed by the type declaration, and no space before the colon.
The colon and the return type declaration have to be on the same line as the argument list closing parenthesis.
Valid: A single space between the colon and type in a return type declaration. | Invalid: No space between the colon and the type in a return type declaration. |
---|---|
|
|
Valid: No space before the colon in a return type declaration. | Invalid: One or more spaces before the colon in a return type declaration. |
---|---|
|
|
PSR12.Keywords.ShortFormTypeKeywords
| [ref]
Short form of type keywords MUST be used i.e. bool instead of boolean, int instead of integer etc.
Valid: Short form type used. | Invalid: Long form type type used. |
---|---|
|
|
PSR12.Traits.UseDeclaration
| [ref]
Squiz.Classes.SelfMemberReference
| [ref]
The self keyword should be used instead of the current class name, should be lowercase, and should not have spaces before or after it.
Valid: Lowercase self used. | Invalid: Uppercase self used. |
---|---|
|
|
Valid: Correct spacing used. | Invalid: Incorrect spacing used. |
---|---|
|
|
Valid: Self used as reference. | Invalid: Local class name used as reference. |
---|---|
|
|
Squiz.Commenting.BlockComment
| [ref]
Squiz.Commenting.ClassComment
| [ref]
Squiz.Commenting.ClosingDeclarationComment
| [ref]
Squiz.Commenting.DocCommentAlignment
| [ref]
The asterisks in a doc comment should align, and there should be one space between the asterisk and tags.
Valid: Asterisks are aligned. | Invalid: Asterisks are not aligned. |
---|---|
|
|
Valid: One space between asterisk and tag. | Invalid: Incorrect spacing used. |
---|---|
|
|
Squiz.Commenting.EmptyCatchComment
| [ref]
Squiz.Commenting.FileComment
| [ref]
Squiz.Commenting.FunctionComment
| [ref]
Squiz.Commenting.FunctionCommentThrowTag
| [ref]
If a function throws any exceptions, they should be documented in a @throws tag.
Valid: @throws tag used. | Invalid: No @throws tag used for throwing function. |
---|---|
|
|
Squiz.Commenting.InlineComment
| [ref]
Squiz.Commenting.VariableComment
| [ref]
Squiz.ControlStructures.ControlSignature
| [ref]
Squiz.Functions.FunctionDeclarationArgumentSpacing
| [ref]
Squiz.Functions.FunctionDuplicateArgument
| [ref]
All PHP built-in functions should be lowercased when called.
Valid: Lowercase function call. | Invalid: isset not called as lowercase. |
---|---|
|
|
Squiz.Functions.MultiLineFunctionDeclaration
| [ref]
Squiz.Operators.IncrementDecrementUsage
| [ref]
Squiz.Operators.ValidLogicalOperators
| [ref]
Squiz.PHP.CommentedOutCode
| [ref]
Squiz.PHP.DisallowMultipleAssignments
| [ref]
Squiz.PHP.DisallowSizeFunctionsInLoops
| [ref]
Squiz.PHP.EmbeddedPhp
| [ref]
Squiz.PHP.Eval
| [ref]
Squiz.PHP.NonExecutableCode
| [ref]
Squiz.Scope.MethodScope
| [ref]
Squiz.Strings.ConcatenationSpacing
| [ref]
Squiz.Strings.DoubleQuoteUsage
| [ref]
Squiz.WhiteSpace.CastSpacing
| [ref]
Casts should not have whitespace inside the parentheses.
Valid: No spaces. | Invalid: Whitespace used inside parentheses. |
---|---|
|
|
Squiz.WhiteSpace.ScopeKeywordSpacing
| [ref]
The php keywords static, public, private, and protected should have one space after them.
Valid: A single space following the keywords. | Invalid: Multiple spaces following the keywords. |
---|---|
|
|
Squiz.WhiteSpace.SemicolonSpacing
| [ref]
Semicolons should not have spaces before them.
Valid: No space before the semicolon. | Invalid: Space before the semicolon. |
---|---|
|
|
Squiz.WhiteSpace.SuperfluousWhitespace
| [ref]
Universal.Arrays.DisallowShortArraySyntax
| [ref]
The array keyword must be used to define arrays.
Valid: Using long form array syntax. | Invalid: Using short array syntax. |
---|---|
|
|
Universal.Arrays.DuplicateArrayKey
| [ref]
When a second array item with the same key is declared, it will overwrite the first. This sniff detects when this is happening in array declarations.
Valid: Arrays with unique keys. | Invalid: Array with duplicate keys. |
---|---|
|
|
Universal.Classes.ModifierKeywordOrder
| [ref]
Requires that class modifier keywords consistently use the same keyword order.
By default the expected order is "abstract/final readonly", but this can be changed via the sniff configuration.
Valid: Modifier keywords ordered correctly. | Invalid: Modifier keywords in reverse order. |
---|---|
|
|
Universal.Classes.RequireAnonClassParentheses
| [ref]
Require the use of parentheses when declaring an anonymous class.
Valid: Anonymous class with parentheses. | Invalid: Anonymous class without parentheses. |
---|---|
|
|
Universal.CodeAnalysis.ConstructorDestructorReturn
| [ref]
A class constructor/destructor can not have a return type declarations. This would result in a fatal error.
Valid: no return type declaration. | Invalid: return type declaration. |
---|---|
|
|
Valid: class constructor/destructor doesn't return anything. | Invalid: class constructor/destructor returns a value. |
---|---|
|
|
Universal.CodeAnalysis.ForeachUniqueAssignment
| [ref]
When a foreach control structure uses the same variable for both the $key as well as the $value assignment, the key will be disregarded and be inaccessible and the variable will contain the value. Mix in reference assignments and the behaviour becomes even more unpredictable.
This is a coding error. Either use unique variables for the key and the value or don't assign the key.
Valid: using unique variables. | Invalid: using the same variable for both the key as well as the value. |
---|---|
|
|
Universal.CodeAnalysis.NoDoubleNegative
| [ref]
Detects double negation in code, which is effectively the same as a boolean cast, but with a much higher cognitive load.
Valid: using singular negation or a boolean cast. | Invalid: using double negation (or more). |
---|---|
|
|
Universal.CodeAnalysis.NoEchoSprintf
| [ref]
Detects use of echo [v]sprintf(...);
. Use [v]printf()
instead.
Valid: using [v]printf() or echo with anything but [v]sprintf(). | Invalid: using echo [v]sprintf(). |
---|---|
|
|
Universal.CodeAnalysis.StaticInFinalClass
| [ref]
When a class is declared as final, using the static
keyword for late static binding is unnecessary and redundant.
This rule also covers using static
in a comparison with instanceof
, using static
for class instantiations or as a return type.
`self` should be used instead.
This applies to final classes, anonymous classes (final by nature) and enums (final by design).
Valid: Using 'self' in a final OO construct. | Invalid: Using 'static' in a final OO construct. |
---|---|
|
|
Universal.Constants.LowercaseClassResolutionKeyword
| [ref]
The "class" keyword when used for class name resolution, i.e. ::class
, must be in lowercase.
Valid: using lowercase. | Invalid: using uppercase or mixed case. |
---|---|
|
|
Universal.Constants.ModifierKeywordOrder
| [ref]
Requires that constant modifier keywords consistently use the same keyword order.
By default the expected order is "final visibility", but this can be changed via the sniff configuration.
Valid: Modifier keywords ordered correctly. | Invalid: Modifier keywords in reverse order. |
---|---|
|
|
Universal.Constants.UppercaseMagicConstants
| [ref]
The PHP native __...__
magic constant should be in uppercase.
Valid: using uppercase. | Invalid: using lowercase or mixed case. |
---|---|
|
|
Universal.ControlStructures.DisallowLonelyIf
| [ref]
Disallows if
statements as the only statement in an else
block.
If an `if` statement is the only statement in the `else` block, use `elseif` instead.
Valid: use of elseif or if followed by another statement. | Invalid: lonely if in an else block. |
---|---|
|
|
Universal.Files.SeparateFunctionsFromOO
| [ref]
A file should either declare (global/namespaced) functions or declare OO structures, but not both.
Nested function declarations, i.e. functions declared within a function/method will be disregarded for the purposes of this sniff.
The same goes for anonymous classes, closures and arrow functions.
Valid: Files containing only functions or only OO. | Invalid: File containing both OO structure declarations as well as function declarations. |
---|---|
|
|
Universal.Namespaces.DisallowCurlyBraceSyntax
| [ref]
Namespace declarations using the curly brace syntax are not allowed.
Valid: Namespace declaration without braces. | Invalid: Namespace declaration with braces. |
---|---|
|
|
Universal.Namespaces.DisallowDeclarationWithoutName
| [ref]
Namespace declarations without a namespace name are not allowed.
Valid: Named namespace declaration. | Invalid: Namespace declaration without a name (=global namespace). |
---|---|
|
|
Universal.Namespaces.OneDeclarationPerFile
| [ref]
There should be only one namespace declaration per file.
Valid: One namespace declaration in a file. | Invalid: Multiple namespace declarations in a file. |
---|---|
|
|
Universal.NamingConventions.NoReservedKeywordParameterNames
| [ref]
It is recommended not to use reserved keywords as parameter names as this can become confusing when people use them in function calls using named parameters.
Valid: parameter names do not use reserved keywords. | Invalid: parameter names use reserved keywords. |
---|---|
|
|
Universal.Operators.DisallowShortTernary
| [ref]
Using short ternaries is not allowed.
While short ternaries are useful when used correctly, the principle of them is often misunderstood and they are more often than not used incorrectly, leading to hard to debug issues and/or PHP warnings/notices.
Valid: Full ternary. | Invalid: Short ternary. |
---|---|
|
|
Universal.Operators.DisallowStandalonePostIncrementDecrement
| [ref]
In a stand-alone in/decrement statement, pre-in/decrement should always be favoured over post-in/decrement.
This reduces the chance of bugs when code gets moved around.
Valid: Pre-in/decrement in a stand-alone statement. | Invalid: Post-in/decrement in a stand-alone statement. |
---|---|
|
|
Valid: Single in/decrement operator in a stand-alone statement. | Invalid: Multiple in/decrement operators in a stand-alone statement. |
---|---|
|
|
Universal.Operators.StrictComparisons
| [ref]
Using loose comparisons is not allowed.
Loose comparisons will type juggle the values being compared, which often results in bugs.
Valid: Using strict comparisons. | Invalid: Using loose comparisons. |
---|---|
|
|
Universal.Operators.TypeSeparatorSpacing
| [ref]
There should be no spacing around the union type separator or the intersection type separator.
This applies to all locations where type declarations can be used, i.e. property types, parameter types and return types.
Valid: No space around the separators. | Invalid: Space around the separators. |
---|---|
|
|
Universal.PHP.LowercasePHPTag
| [ref]
Enforces that the PHP open tag is lowercase.
Valid: Lowercase open tag. | Invalid: Uppercase open tag. |
---|---|
|
|
Universal.UseStatements.DisallowMixedGroupUse
| [ref]
Disallow group use statements which combine imports for namespace/OO, functions and/or constants in one statement.
Valid: Single type group use statements. | Invalid: Mixed group use statement. |
---|---|
|
|
Universal.UseStatements.KeywordSpacing
| [ref]
Enforce a single space after the use
, function
, const
keywords and both before and after the as
keyword in import use
statements.
Valid: Single space used around keywords. | Invalid: Incorrect spacing used around keywords. |
---|---|
|
|
Universal.UseStatements.LowercaseFunctionConst
| [ref]
function
and const
keywords in import use
statements should be in lowercase.
Valid: Lowercase keywords. | Invalid: Non-lowercase keywords. |
---|---|
|
|
Universal.UseStatements.NoLeadingBackslash
| [ref]
Import use
statements must never begin with a leading backslash as they should always be fully qualified.
Valid: Import use statement without leading backslash. | Invalid: Import use statement with leading backslash. |
---|---|
|
|
Universal.UseStatements.NoUselessAliases
| [ref]
Detects useless aliases for import use statements.
Aliasing something to the same name as the original construct is considered useless.
Note: as OO and function names in PHP are case-insensitive, aliasing to the same name, using a different case is also considered useless.
Valid: Import use statement with an alias to a different name. | Invalid: Import use statement with an alias to the same name. |
---|---|
|
|
Universal.WhiteSpace.AnonClassKeywordSpacing
| [ref]
Checks the spacing between the "class" keyword and the open parenthesis for anonymous classes with parentheses.
The desired amount of spacing is configurable and defaults to no space.
Valid: No space between the class keyword and the open parenthesis. | Invalid: Space between the class keyword and the open parenthesis. |
---|---|
|
|
Universal.WhiteSpace.CommaSpacing
| [ref]
There should be no space before a comma and exactly one space, or a new line, after a comma.
The sniff makes the following exceptions to this rule:
1. A comma preceded or followed by a parenthesis, curly or square bracket.
These will not be flagged to prevent conflicts with sniffs handling spacing around braces.
2. A comma preceded or followed by another comma, like for skipping items in a list assignment.
These will not be flagged.
3. A comma preceded by a non-indented heredoc/nowdoc closer.
In that case, unless the `php_version` config directive is set to a version higher than PHP 7.3.0,
a new line before will be enforced to prevent parse errors on PHP < 7.3.
Valid: Correct spacing. | Invalid: Incorrect spacing. |
---|---|
|
|
Valid: Comma after the code. | Invalid: Comma after a trailing comment. |
---|---|
|
|
Universal.WhiteSpace.DisallowInlineTabs
| [ref]
Spaces must be used for mid-line alignment.
Valid: Spaces used for alignment. | Invalid: Tabs used for alignment. |
---|---|
|
|
Universal.WhiteSpace.PrecisionAlignment
| [ref]
Detects when the indentation is not a multiple of a tab-width, i.e. when precision alignment is used.
Valid: indentation equals (a multiple of) the tab width. | Invalid: precision alignment used, indentation does not equal (a multiple of) the tab width. |
---|---|
|
|
WordPress.Arrays.ArrayDeclarationSpacing
| [ref]
WordPress.Arrays.ArrayIndentation
| [ref]
The array closing bracket indentation should line up with the start of the content on the line containing the array opener.
Valid: Closing bracket lined up correctly | Invalid: Closing bracket lined up incorrectly |
---|---|
|
|
Valid: Correctly indented array | Invalid: Indented incorrectly; harder to read. |
---|---|
|
|
Valid: Subsequent lines are indented correctly. | Invalid: Subsequent items are indented before the first line item. |
---|---|
|
|
Valid: Opener and comma after closer are indented correctly | Invalid: Opener is aligned incorrectly to match the closer. The comma does not align correctly with the array indentation. |
---|---|
|
|
WordPress.Arrays.ArrayKeySpacingRestrictions
| [ref]
When referring to array items, only include a space around the index if it is a variable or the key is concatenated.
Valid: Correct spacing around the index keys | Invalid: Incorrect spacing around the index keys |
---|---|
|
|
WordPress.Arrays.MultipleStatementAlignment
| [ref]
When declaring arrays, there should be one space on either side of a double arrow operator used to assign a value to a key.
Valid: correct spacing between the key and value. | Invalid: No or incorrect spacing between the key and value. |
---|---|
|
|
Valid: Double arrow operators aligned | Invalid: Not aligned; harder to read |
---|---|
|
|
WordPress.CodeAnalysis.AssignmentInTernaryCondition
| [ref]
WordPress.CodeAnalysis.EscapedNotTranslated
| [ref]
Text intended for translation needs to be wrapped in a localization function call. This sniff will help you find instances where text is escaped for output, but no localization function is called, even though an (unexpected) text domain argument is passed to the escape function.
Valid: esc_html__() used to translate and escape. | Invalid: esc_html() used to only escape a string intended to be translated as well. |
---|---|
|
|
WordPress.DateTime.CurrentTimeTimestamp
| [ref]
Don't use current_time() to get a timestamp as it doesn't produce a Unix (UTC) timestamp, but a "WordPress timestamp", i.e. a Unix timestamp with current timezone offset.
Valid: using time() to get a Unix (UTC) timestamp. | Invalid: using current_time() to get a Unix (UTC) timestamp. |
---|---|
|
|
Valid: using current_time() with a non-timestamp format. | Invalid: using current_time() to get a timezone corrected timestamp. |
---|---|
|
|
WordPress.DateTime.RestrictedFunctions
| [ref]
The restricted functions date_default_timezone_set() and date() should not be used. Using the PHP native date_default_timezone_set() function isn't allowed, because WordPress Core needs the default time zone to be set to UTC for timezone calculations using the WP Core API to work correctly.
Valid: Using DateTime object. | Invalid: Using date_default_timezone_set(). |
---|---|
|
|
Valid: Using gmdate(). | Invalid: Using date(). |
---|---|
|
|
WordPress.DB.DirectDatabaseQuery
| [ref]
WordPress.DB.PreparedSQL
| [ref]
WordPress.DB.PreparedSQLPlaceholders
| [ref]
WordPress.DB.RestrictedClasses
| [ref]
WordPress.DB.RestrictedFunctions
| [ref]
WordPress.DB.SlowDBQuery
| [ref]
WordPress.Files.FileName
| [ref]
WordPress.NamingConventions.PrefixAllGlobals
| [ref]
All globals terms must be prefixed with a theme/plugin specific term. Global terms include Namespace names, Class/Interface/Trait/Enum names (when not namespaced), Functions (when not namespaced or in an OO structure), Constants/Variable names declared in the global namespace, and Hook names.
A prefix must be distinct and unique to the plugin/theme, in order to prevent potential conflicts with other plugins/themes and with WordPress itself.
The prefix used for a plugin/theme may be chosen by the developers and should be defined in a custom PHPCS ruleset to allow for this sniff to verify that the prefix is consistently used.
Prefixes will be treated in a case-insensitive manner.
https://github.com/WordPress/WordPress-Coding-Standards/wiki/Customizable-sniff-properties#naming-conventions-prefix-everything-in-the-global-namespace
Valid: Using the prefix ECPT_ | Invalid: non-prefixed code |
---|---|
|
|
Valid: Using the prefix ECPT_ in namespaced code | Invalid: using a non-prefixed namespace |
---|---|
|
|
Valid: Using the prefix mycoolplugin_ | Invalid: Using a WordPress reserved prefix wp_ |
---|---|
|
|
Valid: Using the distinct prefix MyPlugin | Invalid: Using a two-letter prefix My |
---|---|
|
|
WordPress.NamingConventions.ValidFunctionName
| [ref]
WordPress.NamingConventions.ValidHookName
| [ref]
Use lowercase letters in action and filter names. Separate words using underscores.
Valid: lowercase hook name. | Invalid: mixed case hook name. |
---|---|
|
|
Valid: words separated by underscores. | Invalid: using non-underscore characters to separate words. |
---|---|
|
|
WordPress.NamingConventions.ValidPostTypeSlug
| [ref]
The post type slug used in register_post_type() must be between 1 and 20 characters.
Valid: short post type slug. | Invalid: too long post type slug. |
---|---|
|
|
Valid: no special characters in post type slug. | Invalid: invalid characters in post type slug. |
---|---|
|
|
Valid: static post type slug. | Invalid: dynamic post type slug. |
---|---|
|
|
Valid: prefixed post slug. | Invalid: using a reserved keyword as slug. |
---|---|
|
|
Valid: custom prefix post slug. | Invalid: using a reserved prefix. |
---|---|
|
|
WordPress.NamingConventions.ValidVariableName
| [ref]
WordPress.PHP.DevelopmentFunctions
| [ref]
WordPress.PHP.DiscouragedPHPFunctions
| [ref]
WordPress.PHP.DontExtract
| [ref]
WordPress.PHP.IniSet
| [ref]
Using ini_set() and similar functions for altering PHP settings at runtime is discouraged. Changing runtime configuration might break other plugins and themes, and even WordPress itself.
Valid: ini_set() for a possibly breaking setting. | Invalid: ini_set() for a possibly breaking setting. |
---|---|
|
|
Valid: WordPress functional alternative. | Invalid: ini_set() to alter memory limits. |
---|---|
|
|
WordPress.PHP.NoSilencedErrors
| [ref]
WordPress.PHP.POSIXFunctions
| [ref]
WordPress.PHP.PregQuoteDelimiter
| [ref]
WordPress.PHP.RestrictedPHPFunctions
| [ref]
WordPress.PHP.StrictInArray
| [ref]
When using functions which compare a value to a range of values in an array, make sure a strict comparison is executed.
Typically, this rule verifies function calls to the PHP native `in_array()`, `array_search()` and `array_keys()` functions pass the `$strict` parameter.
Valid: calling in_array() with the $strict parameter set to `true`. | Invalid: calling in_array() without passing the $strict parameter. |
---|---|
|
|
Valid: calling array_search() with the $strict parameter set to `true`. | Invalid: calling array_search() without passing the $strict parameter. |
---|---|
|
|
Valid: calling array_keys() with a $search_value and the $strict parameter set to `true`. | Invalid: calling array_keys() with a $search_value without passing the $strict parameter. |
---|---|
|
|
WordPress.PHP.TypeCasts
| [ref]
WordPress.PHP.YodaConditions
| [ref]
When doing logical comparisons involving variables, the variable must be placed on the right side. All constants, literals, and function calls must be placed on the left side. If neither side is a variable, the order is unimportant.
Valid: The variable is placed on the right | Invalid: The variable has been placed on the left |
---|---|
|
|
WordPress.Security.EscapeOutput
| [ref]
WordPress.Security.NonceVerification
| [ref]
WordPress.Security.PluginMenuSlug
| [ref]
WordPress.Security.SafeRedirect
| [ref]
wp_safe_redirect() should be used whenever possible to prevent open redirect vulnerabilities. One of the main uses of an open redirect vulnerability is to make phishing attacks more credible. In this case the user sees your (trusted) domain and might get redirected to an attacker controlled website aimed at stealing private information.
Valid: Redirect can only go to allowed domains. | Invalid: Unsafe redirect, can be abused. |
---|---|
|
|
WordPress.Security.ValidatedSanitizedInput
| [ref]
WordPress.Utils.I18nTextDomainFixer
| [ref]
WordPress.WhiteSpace.CastStructureSpacing
| [ref]
A type cast should be preceded by whitespace. There is only one exception to this rule: when the cast is preceded by the spread operator there should be no space between the spread operator and the cast.
Valid: space before typecast. | Invalid: no space before typecast. |
---|---|
|
|
WordPress.WhiteSpace.ControlStructureSpacing
| [ref]
Put one space on both sides of the opening and closing parentheses of control structures.
Valid: One space on each side of the open and close parentheses. | Invalid: Incorrect spacing around the open and close parentheses. |
---|---|
|
|
Valid: Open brace on the same line as the keyword/close parenthesis. | Invalid: Open brace on a different line than the keyword/close parenthesis. |
---|---|
|
|
Valid: One space between the keyword/close parenthesis and the open brace. | Invalid: Too much space between the keyword/close parenthesis and the open brace. |
---|---|
|
|
Valid: One space before the colon. | Invalid: No space before the colon. |
---|---|
|
|
Valid: No blank line between the consecutive close braces. | Invalid: Blank line(s) between the consecutive close braces. |
---|---|
|
|
Valid: No blank lines at the start or end of the control structure body. | Invalid: Blank line(s) at the start and end of the control structure body. |
---|---|
|
|
WordPress.WhiteSpace.ObjectOperatorSpacing
| [ref]
The object operators (->, ?->, ::) should not have any spaces around them, though new lines are allowed except for use with the ::class
constant.
Valid: No spaces around the object operator. | Invalid: Whitespace surrounding the object operator. |
---|---|
|
|
WordPress.WhiteSpace.OperatorSpacing
| [ref]
Always put one space on both sides of logical, comparison and concatenation operators. Always put one space after an assignment operator.
Valid: one space before and after an operator. | Invalid: too much/little space. |
---|---|
|
|
Valid: a new line instead of a space is okay too. | Invalid: too much space after operator on new line. |
---|---|
|
|
Valid: one space after assignment operator. | Invalid: too much/little space after assignment operator. |
---|---|
|
|
WordPress.WP.AlternativeFunctions
| [ref]
WordPress.WP.Capabilities
| [ref]
Capabilities passed should be valid capabilities (custom capabilities can be added in the ruleset).
Valid: a WP native or registered custom user capability is used. | Invalid: unknown/unsupported user capability is used. |
---|---|
|
|
Valid: user capability is used. | Invalid: user role is used instead of a capability. |
---|---|
|
|
Valid: a WP native or registered custom user capability is used. | Invalid: deprecated user capability is used. |
---|---|
|
|
WordPress.WP.CapitalPDangit
| [ref]
The correct spelling of "WordPress" should be used in text strings, comments and object names.
In select cases, when part of an identifier or a URL, WordPress does not have to be capitalized.
Valid: WordPress is correctly capitalized. | Invalid: WordPress is not correctly capitalized. |
---|---|
|
|
WordPress.WP.ClassNameCase
| [ref]
It is strongly recommended to refer to WP native classes by their properly cased name.
Valid: reference to a WordPress native class name using the correct case. | Invalid: reference to a WordPress native class name not using the correct case. |
---|---|
|
|
WordPress.WP.CronInterval
| [ref]
Cron schedules running more often than once every 15 minutes are discouraged. Crons running that frequently can negatively impact the performance of a site.
Valid: Cron schedule is created to run once every hour. | Invalid: Cron schedule is added to run more than once per 15 minutes. |
---|---|
|
|
WordPress.WP.DeprecatedClasses
| [ref]
Please refrain from using deprecated WordPress classes.
Valid: use of a current (non-deprecated) class. | Invalid: use of a deprecated class. |
---|---|
|
|
WordPress.WP.DeprecatedFunctions
| [ref]
Please refrain from using deprecated WordPress functions.
Valid: use of a current (non-deprecated) function. | Invalid: use of a deprecated function. |
---|---|
|
|
WordPress.WP.DeprecatedParameters
| [ref]
Please refrain from passing deprecated WordPress function parameters. In case, you need to pass an optional parameter positioned after the deprecated parameter, only ever pass the default value.
Valid: not passing a deprecated parameter. | Invalid: passing a deprecated parameter. |
---|---|
|
|
Valid: passing default value for a deprecated parameter. | Invalid: not passing the default value for a deprecated parameter. |
---|---|
|
|
WordPress.WP.DeprecatedParameterValues
| [ref]
Please refrain from using deprecated WordPress function parameter values.
Valid: passing a valid function parameter value. | Invalid: passing a deprecated function parameter value. |
---|---|
|
|
WordPress.WP.DiscouragedConstants
| [ref]
WordPress.WP.DiscouragedFunctions
| [ref]
WordPress.WP.EnqueuedResourceParameters
| [ref]
The resource version must be set, to prevent the browser from using an outdated, cached version after the resource has been updated.
Valid: Resource has a version number. | Invalid: Resource has no version number set. |
---|---|
|
|
Valid: Resource has a version number. | Invalid: Resource has version set to false. |
---|---|
|
|
Loading scripts in the header blocks parsing of the page and has a negative impact on load times. However, loading in the footer may break compatibility when other scripts rely on the resource to be available at any time.
In that case, you can pass `false` to make it explicit that the script should be loaded in the header of the page.
Valid: The resource is specified to load in the footer. | Invalid: The location to load this resource is not explicitly set. |
---|---|
|
|
WordPress.WP.EnqueuedResources
| [ref]
Scripts must be registered/enqueued via wp_enqueue_script().
Valid: Script registered and enqueued correctly. | Invalid: Script is directly embedded in HTML. |
---|---|
|
|
Valid: Stylesheet registered and enqueued correctly. | Invalid: Stylesheet is directly embedded in HTML. |
---|---|
|
|
WordPress.WP.GlobalVariablesOverride
| [ref]
WordPress.WP.I18n
| [ref]
WordPress.WP.PostsPerPage
| [ref]
Using "posts_per_page" or "numberposts" with the value set to an high number opens up the potential for making requests slow if the query ends up querying thousands of posts.
You should always fetch the lowest number possible that still gives you the number of results you find acceptable.
Valid: posts_per_page is not over limit (default 100). | Invalid: posts_per_page is over limit (default 100). |
---|---|
|
|
Valid: numberposts is not over limit (default 100). | Invalid: numberposts is over limit (default 100). |
---|---|
|
|
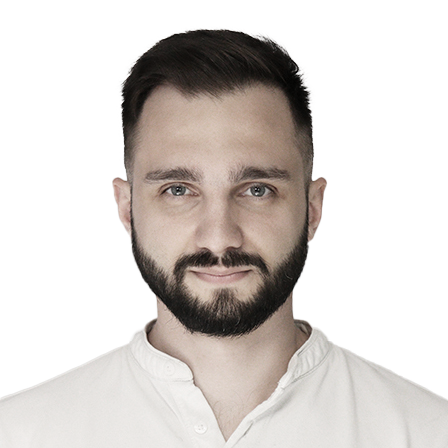
Looking for a developer who
truly cares about your business?
My team and I provide expert consultations, top-notch coding, and comprehensive audits to elevate your success.
Feedback
How satisfied you are after reading this article?