PHPCS: Squiz
Personal documentation for the sniffs available in the PHPCS Squiz Coding Standards to help build an own rulesets and search for error explanations.
Generic.Arrays.ArrayIndent
| [ref]
Generic.Arrays.DisallowLongArraySyntax
| [ref]
Short array syntax must be used to define arrays.
Valid: Short form of array. | Invalid: Long form of array. |
---|---|
|
|
Generic.CodeAnalysis.EmptyStatement
| [ref]
Control Structures must have at least one statement inside of the body.
Valid: There is a statement inside the control structure. | Invalid: The control structure has no statements. |
---|---|
|
|
Generic.Commenting.DocComment
| [ref]
Generic.Commenting.Todo
| [ref]
TODO Statements should be taken care of.
Valid: A comment without a todo. | Invalid: A todo comment. |
---|---|
|
|
Generic.ControlStructures.InlineControlStructure
| [ref]
Control Structures should use braces.
Valid: Braces are used around the control structure. | Invalid: No braces are used for the control structure.. |
---|---|
|
|
Generic.Debug.ClosureLinter
| [ref]
All javascript files should pass basic Closure Linter tests.
Valid: Valid JS Syntax is used. | Invalid: Trailing comma in a javascript array. |
---|---|
|
|
Generic.Files.LineEndings
| [ref]
Unix-style line endings are preferred ("\n" instead of "\r\n").
Generic.Files.LineLength
| [ref]
It is recommended to keep lines at approximately 80 characters long for better code readability.
Generic.Formatting.DisallowMultipleStatements
| [ref]
Multiple statements are not allowed on a single line.
Valid: Two statements are spread out on two separate lines. | Invalid: Two statements are combined onto one line. |
---|---|
|
|
Generic.Formatting.MultipleStatementAlignment
| [ref]
There should be one space on either side of an equals sign used to assign a value to a variable. In the case of a block of related assignments, more space may be inserted to promote readability.
Equals signs aligned | Not aligned; harder to read |
---|---|
|
|
Equals signs aligned; only one space after longest var name | Two spaces after longest var name |
---|---|
|
|
Equals signs aligned | Equals signs not aligned |
---|---|
|
|
Generic.Formatting.SpaceAfterCast
| [ref]
Exactly one space is allowed after a cast.
Valid: A cast operator is followed by one space. | Invalid: A cast operator is not followed by whitespace. |
---|---|
|
|
Generic.Functions.FunctionCallArgumentSpacing
| [ref]
Function arguments should have one space after a comma, and single spaces surrounding the equals sign for default values.
Valid: Single spaces after a comma. | Invalid: No spaces after a comma. |
---|---|
|
|
Valid: Single spaces around an equals sign in function declaration. | Invalid: No spaces around an equals sign in function declaration. |
---|---|
|
|
Generic.NamingConventions.ConstructorName
| [ref]
Constructors should be named __construct, not after the class.
Valid: The constructor is named __construct. | Invalid: The old style class name constructor is used. |
---|---|
|
|
Generic.NamingConventions.UpperCaseConstantName
| [ref]
Constants should always be all-uppercase, with underscores to separate words.
Valid: all uppercase | Invalid: mixed case |
---|---|
|
|
Generic.PHP.DeprecatedFunctions
| [ref]
Deprecated functions should not be used.
Valid: A non-deprecated function is used. | Invalid: A deprecated function is used. |
---|---|
|
|
Generic.PHP.DisallowShortOpenTag
| [ref]
Always use <?php ?> to delimit PHP code, not the <? ?> shorthand. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.ForbiddenFunctions
| [ref]
The forbidden functions sizeof() and delete() should not be used.
Valid: count() is used in place of sizeof(). | Invalid: sizeof() is used. |
---|---|
|
|
Generic.PHP.LowerCaseConstant
| [ref]
The true, false and null constants must always be lowercase.
Valid: lowercase constants | Invalid: uppercase constants |
---|---|
|
|
Generic.PHP.LowerCaseKeyword
| [ref]
All PHP keywords should be lowercase.
Valid: Lowercase array keyword used. | Invalid: Non-lowercase array keyword used. |
---|---|
|
|
Generic.Strings.UnnecessaryStringConcat
| [ref]
Strings should not be concatenated together.
Valid: A string can be concatenated with an expression. | Invalid: Strings should not be concatenated together. |
---|---|
|
|
Generic.WhiteSpace.DisallowTabIndent
| [ref]
Spaces should be used for indentation instead of tabs.
Generic.WhiteSpace.IncrementDecrementSpacing
| [ref]
Generic.WhiteSpace.LanguageConstructSpacing
| [ref]
Language constructs that can be used without parentheses, must have a single space between the language construct keyword and its content.
Valid: Single space after language construct. | Invalid: No space, more than one space or newline after language construct. |
---|---|
|
|
Valid: Single space between yield and from. | Invalid: No space, more than one space or newline between yield and from. |
---|---|
|
|
Generic.WhiteSpace.ScopeIndent
| [ref]
Indentation for control structures, classes, and functions should be 4 spaces per level.
Valid: 4 spaces are used to indent a control structure. | Invalid: 8 spaces are used to indent a control structure. |
---|---|
|
|
PEAR.ControlStructures.MultiLineCondition
| [ref]
Multi-line if conditions should be indented one level and each line should begin with a boolean operator. The end parenthesis should be on a new line.
Valid: Correct indentation. | Invalid: No indentation used on the condition lines. |
---|---|
|
|
Valid: Boolean operator at the start of the line. | Invalid: Boolean operator at the end of the line. |
---|---|
|
|
Valid: End parenthesis on a new line. | Invalid: End parenthesis not moved to a new line. |
---|---|
|
|
PEAR.Files.IncludingFile
| [ref]
Anywhere you are unconditionally including a class file, use require_once. Anywhere you are conditionally including a class file (for example, factory methods), use include_once. Either of these will ensure that class files are included only once. They share the same file list, so you don't need to worry about mixing them - a file included with require_once will not be included again by include_once. Note that include_once and require_once are statements, not functions. Parentheses should not surround the subject filename.
Valid: used as statement | Invalid: used as function |
---|---|
|
|
PEAR.Formatting.MultiLineAssignment
| [ref]
Multi-line assignment should have the equals sign be the first item on the second line indented correctly.
Valid: Assignment operator at the start of the second line. | Invalid: Assignment operator at end of first line. |
---|---|
|
|
Valid: Assignment operator indented one level. | Invalid: Assignment operator not indented. |
---|---|
|
|
PEAR.Functions.FunctionCallSignature
| [ref]
Functions should be called with no spaces between the function name, the opening parenthesis, and the first parameter; and no space between the last parameter, the closing parenthesis, and the semicolon.
Valid: spaces between parameters | Invalid: additional spaces used |
---|---|
|
|
PEAR.Functions.ValidDefaultValue
| [ref]
Arguments with default values go at the end of the argument list.
Valid: argument with default value at end of declaration | Invalid: argument with default value at start of declaration |
---|---|
|
|
PSR2.Classes.PropertyDeclaration
| [ref]
Property names should not be prefixed with an underscore to indicate visibility. Visibility should be used to declare properties rather than the var keyword. Only one property should be declared within a statement. The static declaration must come after the visibility declaration.
Valid: Correct property naming. | Invalid: An underscore prefix used to indicate visibility. |
---|---|
|
|
Valid: Visibility of property declared. | Invalid: Var keyword used to declare property. |
---|---|
|
|
Valid: One property declared per statement. | Invalid: Multiple properties declared in one statement. |
---|---|
|
|
Valid: If declared as static, the static declaration must come after the visibility declaration. | Invalid: Static declaration before the visibility declaration. |
---|---|
|
|
PSR2.Files.EndFileNewline
| [ref]
PHP Files should end with exactly one newline.
PSR2.Methods.MethodDeclaration
| [ref]
Method names should not be prefixed with an underscore to indicate visibility. The static keyword, when present, should come after the visibility declaration, and the final and abstract keywords should come before.
Valid: Correct method naming. | Invalid: An underscore prefix used to indicate visibility. |
---|---|
|
|
Valid: Correct ordering of method prefixes. | Invalid: static keyword used before visibility and final used after. |
---|---|
|
|
Squiz.Arrays.ArrayBracketSpacing
| [ref]
When referencing arrays you should not put whitespace around the opening bracket or before the closing bracket.
Valid: No spaces around the brackets. | Invalid: Spaces around the brackets. |
---|---|
|
|
Squiz.Arrays.ArrayDeclaration
| [ref]
This standard covers all array declarations, regardless of the number and type of values contained within the array. The array keyword must be lowercase.
Valid: array keyword lowercase | Invalid: first letter capitalised |
---|---|
|
|
Valid: first key on second line | Invalid: first key on same line |
---|---|
|
|
Valid: aligned correctly | Invalid: keys and parenthesis aligned incorrectly |
---|---|
|
|
Valid: keys and values aligned | Invalid: alignment incorrect |
---|---|
|
|
Valid: comma after each value | Invalid: no comma after last value |
---|---|
|
|
Squiz.Classes.ClassDeclaration
| [ref]
Squiz.Classes.ClassFileName
| [ref]
Squiz.Classes.DuplicateProperty
| [ref]
Squiz.Classes.LowercaseClassKeywords
| [ref]
The php keywords class, interface, trait, extends, implements, abstract, final, var, and const should be lowercase.
Valid: Lowercase class keywords. | Invalid: Initial capitalization of class keywords. |
---|---|
|
|
Squiz.Classes.SelfMemberReference
| [ref]
The self keyword should be used instead of the current class name, should be lowercase, and should not have spaces before or after it.
Valid: Lowercase self used. | Invalid: Uppercase self used. |
---|---|
|
|
Valid: Correct spacing used. | Invalid: Incorrect spacing used. |
---|---|
|
|
Valid: Self used as reference. | Invalid: Local class name used as reference. |
---|---|
|
|
Squiz.Classes.ValidClassName
| [ref]
Squiz.Commenting.BlockComment
| [ref]
Squiz.Commenting.ClassComment
| [ref]
Squiz.Commenting.ClosingDeclarationComment
| [ref]
Squiz.Commenting.DocCommentAlignment
| [ref]
The asterisks in a doc comment should align, and there should be one space between the asterisk and tags.
Valid: Asterisks are aligned. | Invalid: Asterisks are not aligned. |
---|---|
|
|
Valid: One space between asterisk and tag. | Invalid: Incorrect spacing used. |
---|---|
|
|
Squiz.Commenting.EmptyCatchComment
| [ref]
Squiz.Commenting.FileComment
| [ref]
Squiz.Commenting.FunctionComment
| [ref]
Squiz.Commenting.FunctionCommentThrowTag
| [ref]
If a function throws any exceptions, they should be documented in a @throws tag.
Valid: @throws tag used. | Invalid: No @throws tag used for throwing function. |
---|---|
|
|
Squiz.Commenting.InlineComment
| [ref]
Squiz.Commenting.LongConditionClosingComment
| [ref]
Squiz.Commenting.PostStatementComment
| [ref]
Squiz.Commenting.VariableComment
| [ref]
Squiz.ControlStructures.ControlSignature
| [ref]
Squiz.ControlStructures.ElseIfDeclaration
| [ref]
Squiz.ControlStructures.ForEachLoopDeclaration
| [ref]
There should be a space between each element of a foreach loop and the as keyword should be lowercase.
Valid: Correct spacing used. | Invalid: Invalid spacing used. |
---|---|
|
|
Valid: Lowercase as keyword. | Invalid: Uppercase as keyword. |
---|---|
|
|
Squiz.ControlStructures.ForLoopDeclaration
| [ref]
In a for loop declaration, there should be no space inside the brackets and there should be 0 spaces before and 1 space after semicolons.
Valid: Correct spacing used. | Invalid: Invalid spacing used inside brackets. |
---|---|
|
|
Valid: Correct spacing used. | Invalid: Invalid spacing used before semicolons. |
---|---|
|
|
Valid: Correct spacing used. | Invalid: Invalid spacing used after semicolons. |
---|---|
|
|
Squiz.ControlStructures.InlineIfDeclaration
| [ref]
Squiz.ControlStructures.LowercaseDeclaration
| [ref]
The php keywords if, else, elseif, foreach, for, do, switch, while, try, and catch should be lowercase.
Valid: Lowercase if keyword. | Invalid: Uppercase if keyword. |
---|---|
|
|
Squiz.ControlStructures.SwitchDeclaration
| [ref]
Squiz.CSS.ClassDefinitionClosingBraceSpace
| [ref]
Squiz.CSS.ClassDefinitionNameSpacing
| [ref]
Squiz.CSS.ClassDefinitionOpeningBraceSpace
| [ref]
Squiz.CSS.ColonSpacing
| [ref]
Squiz.CSS.ColourDefinition
| [ref]
Squiz.CSS.DisallowMultipleStyleDefinitions
| [ref]
Squiz.CSS.DuplicateClassDefinition
| [ref]
Squiz.CSS.DuplicateStyleDefinition
| [ref]
Squiz.CSS.EmptyClassDefinition
| [ref]
Squiz.CSS.EmptyStyleDefinition
| [ref]
Squiz.CSS.ForbiddenStyles
| [ref]
Squiz.CSS.Indentation
| [ref]
Squiz.CSS.LowercaseStyleDefinition
| [ref]
Squiz.CSS.MissingColon
| [ref]
Squiz.CSS.NamedColours
| [ref]
Squiz.CSS.Opacity
| [ref]
Squiz.CSS.SemicolonSpacing
| [ref]
Squiz.CSS.ShorthandSize
| [ref]
Squiz.Debug.JavaScriptLint
| [ref]
Squiz.Debug.JSLint
| [ref]
Squiz.Files.FileExtension
| [ref]
Squiz.Formatting.OperatorBracket
| [ref]
Squiz.Functions.FunctionDeclaration
| [ref]
Squiz.Functions.FunctionDeclarationArgumentSpacing
| [ref]
Squiz.Functions.FunctionDuplicateArgument
| [ref]
All PHP built-in functions should be lowercased when called.
Valid: Lowercase function call. | Invalid: isset not called as lowercase. |
---|---|
|
|
Squiz.Functions.GlobalFunction
| [ref]
Squiz.Functions.LowercaseFunctionKeywords
| [ref]
The php keywords function, public, private, protected, and static should be lowercase.
Valid: Lowercase function keyword. | Invalid: Uppercase function keyword. |
---|---|
|
|
Squiz.Functions.MultiLineFunctionDeclaration
| [ref]
Squiz.NamingConventions.ValidFunctionName
| [ref]
Squiz.NamingConventions.ValidVariableName
| [ref]
Squiz.Objects.DisallowObjectStringIndex
| [ref]
Squiz.Objects.ObjectInstantiation
| [ref]
Squiz.Objects.ObjectMemberComma
| [ref]
Squiz.Operators.ComparisonOperatorUsage
| [ref]
Squiz.Operators.IncrementDecrementUsage
| [ref]
Squiz.Operators.ValidLogicalOperators
| [ref]
Squiz.PHP.CommentedOutCode
| [ref]
Squiz.PHP.DisallowBooleanStatement
| [ref]
Squiz.PHP.DisallowComparisonAssignment
| [ref]
Squiz.PHP.DisallowInlineIf
| [ref]
Squiz.PHP.DisallowMultipleAssignments
| [ref]
Squiz.PHP.DisallowSizeFunctionsInLoops
| [ref]
Squiz.PHP.DiscouragedFunctions
| [ref]
Squiz.PHP.EmbeddedPhp
| [ref]
Squiz.PHP.Eval
| [ref]
Squiz.PHP.GlobalKeyword
| [ref]
Squiz.PHP.Heredoc
| [ref]
Squiz.PHP.InnerFunctions
| [ref]
Squiz.PHP.LowercasePHPFunctions
| [ref]
Squiz.PHP.NonExecutableCode
| [ref]
Squiz.Scope.MemberVarScope
| [ref]
Squiz.Scope.MethodScope
| [ref]
Squiz.Scope.StaticThisUsage
| [ref]
Static methods should not use $this.
Valid: Using self:: to access static variables. | Invalid: Using $this-> to access static variables. |
---|---|
|
|
Squiz.Strings.ConcatenationSpacing
| [ref]
Squiz.Strings.DoubleQuoteUsage
| [ref]
Squiz.Strings.EchoedStrings
| [ref]
Simple strings should not be enclosed in parentheses when being echoed.
Valid: Using echo without parentheses. | Invalid: Using echo with parentheses. |
---|---|
|
|
Squiz.WhiteSpace.CastSpacing
| [ref]
Casts should not have whitespace inside the parentheses.
Valid: No spaces. | Invalid: Whitespace used inside parentheses. |
---|---|
|
|
Squiz.WhiteSpace.ControlStructureSpacing
| [ref]
Squiz.WhiteSpace.FunctionClosingBraceSpace
| [ref]
Squiz.WhiteSpace.FunctionOpeningBraceSpace
| [ref]
Squiz.WhiteSpace.FunctionSpacing
| [ref]
Squiz.WhiteSpace.LanguageConstructSpacing
| [ref]
The php constructs echo, print, return, include, include_once, require, require_once, and new should have one space after them.
Valid: echo statement with a single space after it. | Invalid: echo statement with no space after it. |
---|---|
|
|
Squiz.WhiteSpace.LogicalOperatorSpacing
| [ref]
Squiz.WhiteSpace.MemberVarSpacing
| [ref]
Squiz.WhiteSpace.ObjectOperatorSpacing
| [ref]
The object operator (->) should not have any space around it.
Valid: No spaces around the object operator. | Invalid: Whitespace surrounding the object operator. |
---|---|
|
|
Squiz.WhiteSpace.OperatorSpacing
| [ref]
Squiz.WhiteSpace.PropertyLabelSpacing
| [ref]
Squiz.WhiteSpace.ScopeClosingBrace
| [ref]
Squiz.WhiteSpace.ScopeKeywordSpacing
| [ref]
The php keywords static, public, private, and protected should have one space after them.
Valid: A single space following the keywords. | Invalid: Multiple spaces following the keywords. |
---|---|
|
|
Squiz.WhiteSpace.SemicolonSpacing
| [ref]
Semicolons should not have spaces before them.
Valid: No space before the semicolon. | Invalid: Space before the semicolon. |
---|---|
|
|
Squiz.WhiteSpace.SuperfluousWhitespace
| [ref]
Zend.Debug.CodeAnalyzer
| [ref]
PHP Code should pass the zend code analyzer.
Valid: Valid PHP Code. | Invalid: There is an unused function parameter. |
---|---|
|
|
Zend.Files.ClosingTag
| [ref]
Files should not have closing php tags.
Valid: No closing tag at the end of the file. | Invalid: A closing php tag is included at the end of the file. |
---|---|
|
|
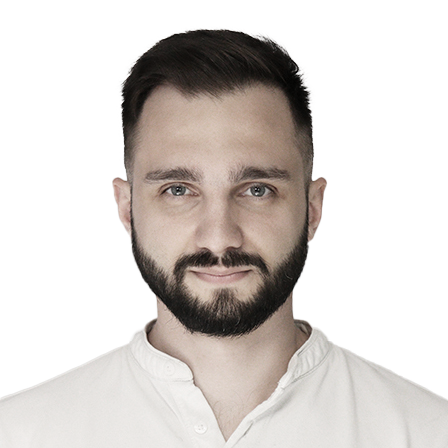
Looking for a developer who
truly cares about your business?
My team and I provide expert consultations, top-notch coding, and comprehensive audits to elevate your success.
Feedback
How satisfied you are after reading this article?