PHPCS: Pear
Personal documentation for the sniffs available in the PHPCS Pear Coding Standards to help build an own rulesets and search for error explanations.
Generic.Commenting.DocComment
| [ref]# PEAR Coding Standard
Generic.ControlStructures.InlineControlStructure
| [ref]# PEAR Coding Standard
Control Structures should use braces.
Valid: Braces are used around the control structure. | Invalid: No braces are used for the control structure.. |
---|---|
|
|
Generic.Files.LineEndings
| [ref]# PEAR Coding Standard
Unix-style line endings are preferred ("\n" instead of "\r\n").
Generic.Files.LineLength
| [ref]# PEAR Coding Standard
It is recommended to keep lines at approximately 80 characters long for better code readability.
Generic.Functions.FunctionCallArgumentSpacing
| [ref]# PEAR Coding Standard
Function arguments should have one space after a comma, and single spaces surrounding the equals sign for default values.
Valid: Single spaces after a comma. | Invalid: No spaces after a comma. |
---|---|
|
|
Valid: Single spaces around an equals sign in function declaration. | Invalid: No spaces around an equals sign in function declaration. |
---|---|
|
|
Generic.NamingConventions.UpperCaseConstantName
| [ref]# PEAR Coding Standard
Constants should always be all-uppercase, with underscores to separate words.
Valid: all uppercase | Invalid: mixed case |
---|---|
|
|
Generic.PHP.DisallowShortOpenTag
| [ref]# PEAR Coding Standard
Always use <?php ?> to delimit PHP code, not the <? ?> shorthand. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.LowerCaseConstant
| [ref]# PEAR Coding Standard
The true, false and null constants must always be lowercase.
Valid: lowercase constants | Invalid: uppercase constants |
---|---|
|
|
Generic.WhiteSpace.DisallowTabIndent
| [ref]# PEAR Coding Standard
Spaces should be used for indentation instead of tabs.
PEAR.Classes.ClassDeclaration
| [ref]# PEAR Coding Standard
The opening brace of a class must be on the line after the definition by itself.
Valid: Opening brace on the correct line. | Invalid: Opening brace on same line as declaration. |
---|---|
|
|
PEAR.Commenting.ClassComment
| [ref]# PEAR Coding Standard
Classes and interfaces must have a non-empty doc comment. The short description must be on the second line of the comment. Each description must have one blank comment line before and after. There must be one blank line before the tags in the comments. A @version tag must be in Release: package_version format.
Valid: A doc comment for the class. | Invalid: No doc comment for the class. |
---|---|
|
|
Valid: A doc comment for the class. | Invalid: Invalid comment type for the class. |
---|---|
|
|
Valid: A doc comment for the class. | Invalid: The blank line after the comment makes it appear as a file comment, not a class comment. |
---|---|
|
|
Valid: Short description is the second line of the comment. | Invalid: An extra blank line before the short description. |
---|---|
|
|
Valid: Exactly one blank line around descriptions. | Invalid: Extra blank lines around the descriptions. |
---|---|
|
|
Valid: Exactly one blank line before the tags. | Invalid: Extra blank lines before the tags. |
---|---|
|
|
Valid: Version tag is in the correct format. | Invalid: No Release: text. |
---|---|
|
|
PEAR.Commenting.FileComment
| [ref]# PEAR Coding Standard
Files must have a non-empty doc comment. The short description must be on the second line of the comment. Each description must have one blank comment line before and after. There must be one blank line before the tags in the comments. There must be a category, package, author, license, and link tag. There may only be one category, package, subpackage, license, version, since and deprecated tag. The tags must be in the order category, package, subpackage, author, copyright, license, version, link, see, since, and deprecated. The php version must be specified.
Valid: A file comment is used. | Invalid: No doc comment for the class. |
---|---|
|
|
Valid: Short description is the second line of the comment. | Invalid: An extra blank line before the short description. |
---|---|
|
|
Valid: Exactly one blank line around descriptions. | Invalid: Extra blank lines around the descriptions. |
---|---|
|
|
Valid: Exactly one blank line before the tags. | Invalid: Extra blank lines before the tags. |
---|---|
|
|
Valid: All required tags are used. | Invalid: Missing an author tag. |
---|---|
|
|
Valid: Tags that should only be used once are only used once. | Invalid: Multiple category tags. |
---|---|
|
|
Valid: PHP version specified. | Invalid: Category and package tags are swapped in order. |
---|---|
|
|
Valid: Tags are in the correct order. | Invalid: No php version specified. |
---|---|
|
|
PEAR.Commenting.FunctionComment
| [ref]# PEAR Coding Standard
Functions must have a non-empty doc comment. The short description must be on the second line of the comment. Each description must have one blank comment line before and after. There must be one blank line before the tags in the comments. There must be a tag for each of the parameters in the right order with the right variable names with a comment. There must be a return tag. Any throw tag must have an exception class.
Valid: A function doc comment is used. | Invalid: No doc comment for the function. |
---|---|
|
|
Valid: Short description is the second line of the comment. | Invalid: An extra blank line before the short description. |
---|---|
|
|
Valid: Exactly one blank line around descriptions. | Invalid: Extra blank lines around the descriptions. |
---|---|
|
|
Valid: Exactly one blank line before the tags. | Invalid: Extra blank lines before the tags. |
---|---|
|
|
Valid: Throws tag has an exception class. | Invalid: No exception class given for throws tag. |
---|---|
|
|
Valid: Return tag present. | Invalid: No return tag. |
---|---|
|
|
Valid: Param names are correct. | Invalid: Wrong parameter name doesn't match function signature. |
---|---|
|
|
Valid: Param names are ordered correctly. | Invalid: Wrong parameter order. |
---|---|
|
|
PEAR.Commenting.InlineComment
| [ref]# PEAR Coding Standard
Perl-style # comments are not allowed.
Valid: A // style comment. | Invalid: A # style comment. |
---|---|
|
|
PEAR.ControlStructures.ControlSignature
| [ref]# PEAR Coding Standard
Control structures should use one space around the parentheses in conditions. The opening brace should be preceded by one space and should be at the end of the line.
Valid: Correct spacing around the condition. | Invalid: Incorrect spacing around the condition. |
---|---|
|
|
Valid: Correct placement of the opening brace. | Invalid: Incorrect placement of the opening brace on a new line. |
---|---|
|
|
PEAR.ControlStructures.MultiLineCondition
| [ref]# PEAR Coding Standard
Multi-line if conditions should be indented one level and each line should begin with a boolean operator. The end parenthesis should be on a new line.
Valid: Correct indentation. | Invalid: No indentation used on the condition lines. |
---|---|
|
|
Valid: Boolean operator at the start of the line. | Invalid: Boolean operator at the end of the line. |
---|---|
|
|
Valid: End parenthesis on a new line. | Invalid: End parenthesis not moved to a new line. |
---|---|
|
|
PEAR.Files.IncludingFile
| [ref]# PEAR Coding Standard
Anywhere you are unconditionally including a class file, use require_once. Anywhere you are conditionally including a class file (for example, factory methods), use include_once. Either of these will ensure that class files are included only once. They share the same file list, so you don't need to worry about mixing them - a file included with require_once will not be included again by include_once. Note that include_once and require_once are statements, not functions. Parentheses should not surround the subject filename.
Valid: used as statement | Invalid: used as function |
---|---|
|
|
PEAR.Formatting.MultiLineAssignment
| [ref]# PEAR Coding Standard
Multi-line assignment should have the equals sign be the first item on the second line indented correctly.
Valid: Assignment operator at the start of the second line. | Invalid: Assignment operator at end of first line. |
---|---|
|
|
Valid: Assignment operator indented one level. | Invalid: Assignment operator not indented. |
---|---|
|
|
PEAR.Functions.FunctionCallSignature
| [ref]# PEAR Coding Standard
Functions should be called with no spaces between the function name, the opening parenthesis, and the first parameter; and no space between the last parameter, the closing parenthesis, and the semicolon.
Valid: spaces between parameters | Invalid: additional spaces used |
---|---|
|
|
PEAR.Functions.FunctionDeclaration
| [ref]# PEAR Coding Standard
There should be exactly 1 space after the function keyword and 1 space on each side of the use keyword. Closures should use the Kernighan/Ritchie Brace style and other single-line functions should use the BSD/Allman style. Multi-line function declarations should have the parameter lists indented one level with the closing parenthesis on a newline followed by a single space and the opening brace of the function.
Valid: Correct spacing around function and use keywords. | Invalid: No spacing around function and use keywords. |
---|---|
|
|
Valid: Multi-line function declaration formatted properly. | Invalid: Invalid indentation and formatting of closing parenthesis. |
---|---|
|
|
PEAR.Functions.ValidDefaultValue
| [ref]# PEAR Coding Standard
Arguments with default values go at the end of the argument list.
Valid: argument with default value at end of declaration | Invalid: argument with default value at start of declaration |
---|---|
|
|
PEAR.NamingConventions.ValidClassName
| [ref]# PEAR Coding Standard
Classes should be given descriptive names. Avoid using abbreviations where possible. Class names should always begin with an uppercase letter. The PEAR class hierarchy is also reflected in the class name, each level of the hierarchy separated with a single underscore.
Examples of valid class names | Examples of invalid class names |
---|---|
|
|
PEAR.NamingConventions.ValidFunctionName
| [ref]# PEAR Coding Standard
Functions and methods should be named using the "studly caps" style (also referred to as "bumpy case" or "camel caps"). Functions should in addition have the package name as a prefix, to avoid name collisions between packages. The initial letter of the name (after the prefix) is lowercase, and each letter that starts a new "word" is capitalized.
Examples of valid function names | Examples of invalid function names |
---|---|
|
|
PEAR.NamingConventions.ValidVariableName
| [ref]# PEAR Coding Standard
Private member variable names should be prefixed with an underscore and public/protected variable names should not.
Valid: Proper member variable names. | Invalid: underscores used on public/protected variables and not used on private variables. |
---|---|
|
|
PEAR.WhiteSpace.ObjectOperatorIndent
| [ref]# PEAR Coding Standard
Chained object operators when spread out over multiple lines should be the first thing on the line and be indented by 1 level.
Valid: Object operator at the start of a new line. | Invalid: Object operator at the end of the line. |
---|---|
|
|
Valid: Object operator indented correctly. | Invalid: Object operator not indented correctly. |
---|---|
|
|
PEAR.WhiteSpace.ScopeClosingBrace
| [ref]# PEAR Coding Standard
Closing braces should be indented at the same level as the beginning of the scope.
Valid: Consistent indentation level for scope. | Invalid: The ending brace is indented further than the if statement. |
---|---|
|
|
PEAR.WhiteSpace.ScopeIndent
| [ref]# PEAR Coding Standard
Any scope openers except for switch statements should be indented 1 level. This includes classes, functions, and control structures.
Valid: Consistent indentation level for scope. | Invalid: Indentation is not used for scope. |
---|---|
|
|
Squiz.Commenting.DocCommentAlignment
| [ref]# PEAR Coding Standard
The asterisks in a doc comment should align, and there should be one space between the asterisk and tags.
Valid: Asterisks are aligned. | Invalid: Asterisks are not aligned. |
---|---|
|
|
Valid: One space between asterisk and tag. | Invalid: Incorrect spacing used. |
---|---|
|
|
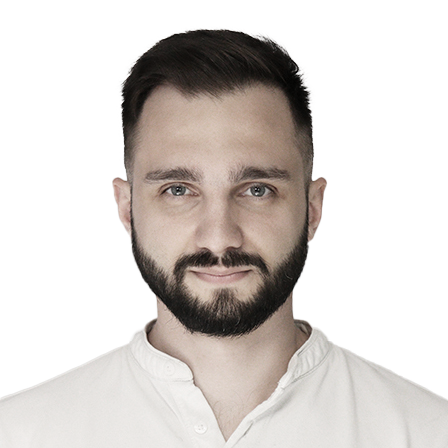
Looking for a developer who
truly cares about your business?
My team and I provide expert consultations, top-notch coding, and comprehensive audits to elevate your success.
Feedback
How satisfied you are after reading this article?