PHPCS: Generic
Personal documentation for the sniffs available in the PHPCS Generic Coding Standards to help build an own rulesets and search for error explanations.
Generic.Arrays.ArrayIndent
| [ref]
Generic.Arrays.DisallowLongArraySyntax
| [ref]
Short array syntax must be used to define arrays.
Valid: Short form of array. | Invalid: Long form of array. |
---|---|
|
|
Generic.Arrays.DisallowShortArraySyntax
| [ref]
Long array syntax must be used to define arrays.
Valid: Long form of array. | Invalid: Short form of array. |
---|---|
|
|
Generic.Classes.DuplicateClassName
| [ref]
Class and Interface names should be unique in a project. They should never be duplicated.
Valid: A unique class name. | Invalid: A class duplicated (including across multiple files). |
---|---|
|
|
Generic.Classes.OpeningBraceSameLine
| [ref]
The opening brace of a class must be on the same line after the definition and must be the last thing on that line.
Valid: Opening brace on the same line. | Invalid: Opening brace on the next line. |
---|---|
|
|
Valid: Opening brace is the last thing on the line. | Invalid: Opening brace not last thing on the line. |
---|---|
|
|
Generic.CodeAnalysis.AssignmentInCondition
| [ref]
Variable assignments should not be made within conditions.
Valid: A variable comparison being executed within a condition. | Invalid: A variable assignment being made within a condition. |
---|---|
|
|
Generic.CodeAnalysis.EmptyPHPStatement
| [ref]
Empty PHP tags are not allowed.
Valid: There is at least one statement inside the PHP tag pair. | Invalid: There is no statement inside the PHP tag pair. |
---|---|
|
|
Valid: There is no superfluous semi-colon after a PHP statement. | Invalid: There are one or more superfluous semi-colons after a PHP statement. |
---|---|
|
|
Generic.CodeAnalysis.EmptyStatement
| [ref]
Control Structures must have at least one statement inside of the body.
Valid: There is a statement inside the control structure. | Invalid: The control structure has no statements. |
---|---|
|
|
Generic.CodeAnalysis.ForLoopShouldBeWhileLoop
| [ref]
For loops that have only a second expression (the condition) should be converted to while loops.
Valid: A for loop is used with all three expressions. | Invalid: A for loop is used without a first or third expression. |
---|---|
|
|
Generic.CodeAnalysis.ForLoopWithTestFunctionCall
| [ref]
For loops should not call functions inside the test for the loop when they can be computed beforehand.
Valid: A for loop that determines its end condition before the loop starts. | Invalid: A for loop that unnecessarily computes the same value on every iteration. |
---|---|
|
|
Generic.CodeAnalysis.JumbledIncrementer
| [ref]
Incrementers in nested loops should use different variable names.
Valid: Two different variables being used to increment. | Invalid: Inner incrementer is the same variable name as the outer one. |
---|---|
|
|
Generic.CodeAnalysis.UnconditionalIfStatement
| [ref]
If statements that are always evaluated should not be used.
Valid: An if statement that only executes conditionally. | Invalid: An if statement that is always performed. |
---|---|
|
|
Valid: An if statement that only executes conditionally. | Invalid: An if statement that is never performed. |
---|---|
|
|
Generic.CodeAnalysis.UnnecessaryFinalModifier
| [ref]
Methods should not be declared final inside of classes that are declared final.
Valid: A method in a final class is not marked final. | Invalid: A method in a final class is also marked final. |
---|---|
|
|
Generic.CodeAnalysis.UnusedFunctionParameter
| [ref]
All parameters in a functions signature should be used within the function.
Valid: All the parameters are used. | Invalid: One of the parameters is not being used. |
---|---|
|
|
Generic.CodeAnalysis.UselessOverridingMethod
| [ref]
Methods should not be defined that only call the parent method.
Valid: A method that extends functionality on a parent method. | Invalid: An overriding method that only calls the parent. |
---|---|
|
|
Generic.Commenting.DocComment
| [ref]
Generic.Commenting.Fixme
| [ref]
FIXME Statements should be taken care of.
Valid: A comment without a fixme. | Invalid: A fixme comment. |
---|---|
|
|
Generic.Commenting.Todo
| [ref]
TODO Statements should be taken care of.
Valid: A comment without a todo. | Invalid: A todo comment. |
---|---|
|
|
Generic.ControlStructures.DisallowYodaConditions
| [ref]
Yoda conditions are disallowed.
Valid: value to be asserted must go on the right side of the comparison. | Invalid: value to be asserted must not be on the left. |
---|---|
|
|
Generic.ControlStructures.InlineControlStructure
| [ref]
Control Structures should use braces.
Valid: Braces are used around the control structure. | Invalid: No braces are used for the control structure.. |
---|---|
|
|
Generic.Debug.ClosureLinter
| [ref]
All javascript files should pass basic Closure Linter tests.
Valid: Valid JS Syntax is used. | Invalid: Trailing comma in a javascript array. |
---|---|
|
|
Generic.Debug.CSSLint
| [ref]
All css files should pass the basic csslint tests.
Valid: Valid CSS Syntax is used. | Invalid: The CSS has a typo in it. |
---|---|
|
|
Generic.Debug.ESLint
| [ref]
Generic.Debug.JSHint
| [ref]
All javascript files should pass basic JSHint tests.
Valid: Valid JS Syntax is used. | Invalid: The Javascript is using an undefined variable. |
---|---|
|
|
Generic.Files.ByteOrderMark
| [ref]
Byte Order Marks that may corrupt your application should not be used. These include 0xefbbbf (UTF-8), 0xfeff (UTF-16 BE) and 0xfffe (UTF-16 LE).
Generic.Files.EndFileNewline
| [ref]
Files should end with a newline character.
Generic.Files.EndFileNoNewline
| [ref]
Files should not end with a newline character.
Generic.Files.ExecutableFile
| [ref]
Files should not be executable.
Generic.Files.InlineHTML
| [ref]
Files that contain php code should only have php code and should not have any "inline html".
Valid: A php file with only php code in it. | Invalid: A php file with html in it outside of the php tags. |
---|---|
|
|
Generic.Files.LineEndings
| [ref]
Unix-style line endings are preferred ("\n" instead of "\r\n").
Generic.Files.LineLength
| [ref]
It is recommended to keep lines at approximately 80 characters long for better code readability.
Generic.Files.LowercasedFilename
| [ref]
Lowercase filenames are required.
Generic.Files.OneClassPerFile
| [ref]
There should only be one class defined in a file.
Valid: Only one class in the file. | Invalid: Multiple classes defined in one file. |
---|---|
|
|
Generic.Files.OneInterfacePerFile
| [ref]
There should only be one interface defined in a file.
Valid: Only one interface in the file. | Invalid: Multiple interfaces defined in one file. |
---|---|
|
|
Generic.Files.OneObjectStructurePerFile
| [ref]
There should only be one class or interface or trait defined in a file.
Valid: Only one object structure in the file. | Invalid: Multiple object structures defined in one file. |
---|---|
|
|
Generic.Files.OneTraitPerFile
| [ref]
There should only be one trait defined in a file.
Valid: Only one trait in the file. | Invalid: Multiple traits defined in one file. |
---|---|
|
|
Generic.Formatting.DisallowMultipleStatements
| [ref]
Multiple statements are not allowed on a single line.
Valid: Two statements are spread out on two separate lines. | Invalid: Two statements are combined onto one line. |
---|---|
|
|
Generic.Formatting.MultipleStatementAlignment
| [ref]
There should be one space on either side of an equals sign used to assign a value to a variable. In the case of a block of related assignments, more space may be inserted to promote readability.
Equals signs aligned | Not aligned; harder to read |
---|---|
|
|
Equals signs aligned; only one space after longest var name | Two spaces after longest var name |
---|---|
|
|
Equals signs aligned | Equals signs not aligned |
---|---|
|
|
Generic.Formatting.NoSpaceAfterCast
| [ref]
Spaces are not allowed after casting operators.
Valid: A cast operator is immediately before its value. | Invalid: A cast operator is followed by whitespace. |
---|---|
|
|
Generic.Formatting.SpaceAfterCast
| [ref]
Exactly one space is allowed after a cast.
Valid: A cast operator is followed by one space. | Invalid: A cast operator is not followed by whitespace. |
---|---|
|
|
Generic.Formatting.SpaceAfterNot
| [ref]
Exactly one space is allowed after the NOT operator.
Valid: A NOT operator followed by one space. | Invalid: A NOT operator not followed by whitespace or followed by too much whitespace. |
---|---|
|
|
Generic.Formatting.SpaceBeforeCast
| [ref]
There should be exactly one space before a cast operator.
Valid: Single space before a cast operator. | Invalid: No space or multiple spaces before a cast operator. |
---|---|
|
|
Generic.Functions.CallTimePassByReference
| [ref]
Call-time pass-by-reference is not allowed. It should be declared in the function definition.
Valid: Pass-by-reference is specified in the function definition. | Invalid: Pass-by-reference is done in the call to a function. |
---|---|
|
|
Generic.Functions.FunctionCallArgumentSpacing
| [ref]
Function arguments should have one space after a comma, and single spaces surrounding the equals sign for default values.
Valid: Single spaces after a comma. | Invalid: No spaces after a comma. |
---|---|
|
|
Valid: Single spaces around an equals sign in function declaration. | Invalid: No spaces around an equals sign in function declaration. |
---|---|
|
|
Generic.Functions.OpeningFunctionBraceBsdAllman
| [ref]
Function declarations follow the "BSD/Allman style". The function brace is on the line following the function declaration and is indented to the same column as the start of the function declaration.
Valid: brace on next line | Invalid: brace on same line |
---|---|
|
|
Generic.Functions.OpeningFunctionBraceKernighanRitchie
| [ref]
Function declarations follow the "Kernighan/Ritchie style". The function brace is on the same line as the function declaration. One space is required between the closing parenthesis and the brace.
Valid: brace on same line | Invalid: brace on next line |
---|---|
|
|
Generic.Metrics.CyclomaticComplexity
| [ref]
Functions should not have a cyclomatic complexity greater than 20, and should try to stay below a complexity of 10.
Generic.Metrics.NestingLevel
| [ref]
Functions should not have a nesting level greater than 10, and should try to stay below 5.
Generic.NamingConventions.AbstractClassNamePrefix
| [ref]
Abstract class names must be prefixed with "Abstract", e.g. AbstractBar.
Valid: | Invalid: |
---|---|
|
|
Generic.NamingConventions.CamelCapsFunctionName
| [ref]
Functions should use camelCaps format for their names. Only PHP's magic methods should use a double underscore prefix.
Valid: A function in camelCaps format. | Invalid: A function in snake_case format. |
---|---|
|
|
Generic.NamingConventions.ConstructorName
| [ref]
Constructors should be named __construct, not after the class.
Valid: The constructor is named __construct. | Invalid: The old style class name constructor is used. |
---|---|
|
|
Generic.NamingConventions.InterfaceNameSuffix
| [ref]
Interface names must be suffixed with "Interface", e.g. BarInterface.
Valid: | Invalid: |
---|---|
|
|
Generic.NamingConventions.TraitNameSuffix
| [ref]
Trait names must be suffixed with "Trait", e.g. BarTrait.
Valid: | Invalid: |
---|---|
|
|
Generic.NamingConventions.UpperCaseConstantName
| [ref]
Constants should always be all-uppercase, with underscores to separate words.
Valid: all uppercase | Invalid: mixed case |
---|---|
|
|
Generic.PHP.BacktickOperator
| [ref]
Disallow the use of the backtick operator for execution of shell commands.
Generic.PHP.CharacterBeforePHPOpeningTag
| [ref]
The opening php tag should be the first item in the file.
Valid: A file starting with an opening php tag. | Invalid: A file with content before the opening php tag. |
---|---|
|
|
Generic.PHP.ClosingPHPTag
| [ref]
All opening php tags should have a corresponding closing tag.
Valid: A closing tag paired with it's opening tag. | Invalid: No closing tag paired with the opening tag. |
---|---|
|
|
Generic.PHP.DeprecatedFunctions
| [ref]
Deprecated functions should not be used.
Valid: A non-deprecated function is used. | Invalid: A deprecated function is used. |
---|---|
|
|
Generic.PHP.DisallowAlternativePHPTags
| [ref]
Always use <?php ?> to delimit PHP code, do not use the ASP <% %> style tags nor the <script language="php"></script> tags. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.DisallowRequestSuperglobal
| [ref]
$_REQUEST should never be used due to the ambiguity created as to where the data is coming from. Use $_POST, $_GET, or $_COOKIE instead.
Generic.PHP.DisallowShortOpenTag
| [ref]
Always use <?php ?> to delimit PHP code, not the <? ?> shorthand. This is the most portable way to include PHP code on differing operating systems and setups.
Generic.PHP.DiscourageGoto
| [ref]
Discourage the use of the PHP goto
language construct.
Generic.PHP.ForbiddenFunctions
| [ref]
The forbidden functions sizeof() and delete() should not be used.
Valid: count() is used in place of sizeof(). | Invalid: sizeof() is used. |
---|---|
|
|
Generic.PHP.LowerCaseConstant
| [ref]
The true, false and null constants must always be lowercase.
Valid: lowercase constants | Invalid: uppercase constants |
---|---|
|
|
Generic.PHP.LowerCaseKeyword
| [ref]
All PHP keywords should be lowercase.
Valid: Lowercase array keyword used. | Invalid: Non-lowercase array keyword used. |
---|---|
|
|
Generic.PHP.LowerCaseType
| [ref]
All PHP types used for parameter type and return type declarations should be lowercase.
Valid: Lowercase type declarations used. | Invalid: Non-lowercase type declarations used. |
---|---|
|
|
Valid: Lowercase type used. | Invalid: Non-lowercase type used. |
---|---|
|
|
Generic.PHP.NoSilencedErrors
| [ref]
Suppressing Errors is not allowed.
Valid: isset() is used to verify that a variable exists before trying to use it. | Invalid: Errors are suppressed. |
---|---|
|
|
Generic.PHP.RequireStrictTypes
| [ref]
Generic.PHP.SAPIUsage
| [ref]
The PHP_SAPI constant should be used instead of php_sapi_name().
Valid: PHP_SAPI is used. | Invalid: php_sapi_name() is used. |
---|---|
|
|
Generic.PHP.Syntax
| [ref]
The code should use valid PHP syntax.
Valid: No PHP syntax errors. | Invalid: Code contains PHP syntax errors. |
---|---|
|
|
Generic.PHP.UpperCaseConstant
| [ref]
The true, false and null constants must always be uppercase.
Valid: uppercase constants | Invalid: lowercase constants |
---|---|
|
|
Generic.Strings.UnnecessaryStringConcat
| [ref]
Strings should not be concatenated together.
Valid: A string can be concatenated with an expression. | Invalid: Strings should not be concatenated together. |
---|---|
|
|
Generic.VersionControl.GitMergeConflict
| [ref]
Generic.VersionControl.SubversionProperties
| [ref]
All php files in a subversion repository should have the svn:keywords property set to 'Author Id Revision' and the svn:eol-style property set to 'native'.
Generic.WhiteSpace.ArbitraryParenthesesSpacing
| [ref]
Arbitrary sets of parentheses should have no spaces inside.
Valid: no spaces on the inside of a set of arbitrary parentheses. | Invalid: spaces or new lines on the inside of a set of arbitrary parentheses. |
---|---|
|
|
Generic.WhiteSpace.DisallowSpaceIndent
| [ref]
Tabs should be used for indentation instead of spaces.
Generic.WhiteSpace.DisallowTabIndent
| [ref]
Spaces should be used for indentation instead of tabs.
Generic.WhiteSpace.IncrementDecrementSpacing
| [ref]
Generic.WhiteSpace.LanguageConstructSpacing
| [ref]
Language constructs that can be used without parentheses, must have a single space between the language construct keyword and its content.
Valid: Single space after language construct. | Invalid: No space, more than one space or newline after language construct. |
---|---|
|
|
Valid: Single space between yield and from. | Invalid: No space, more than one space or newline between yield and from. |
---|---|
|
|
Generic.WhiteSpace.ScopeIndent
| [ref]
Indentation for control structures, classes, and functions should be 4 spaces per level.
Valid: 4 spaces are used to indent a control structure. | Invalid: 8 spaces are used to indent a control structure. |
---|---|
|
|
Generic.WhiteSpace.SpreadOperatorSpacingAfter
| [ref]
There should be no space between the spread operator and the variable/function call it applies to.
Valid: No space between the spread operator and the variable/function call it applies to. | Invalid: space found between the spread operator and the variable/function call it applies to. |
---|---|
|
|
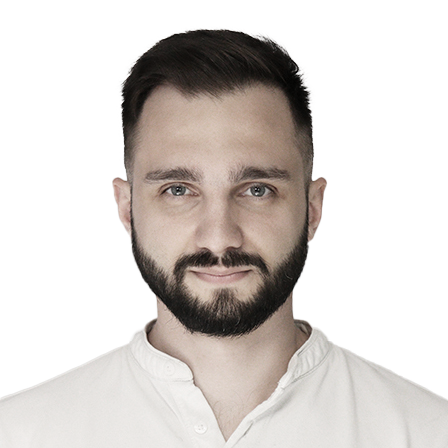
Looking for a developer who
truly cares about your business?
My team and I provide expert consultations, top-notch coding, and comprehensive audits to elevate your success.
Feedback
How satisfied you are after reading this article?